Weather App in R
This script is a Shiny app that allows the user to select a municipality in Latvia from a dropdown menu and view the current weather and temperature forecast for that municipality.
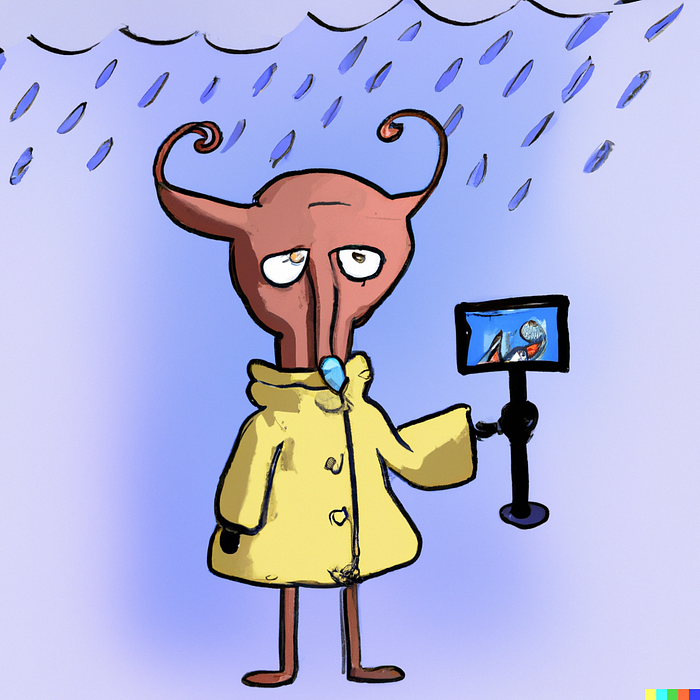
library(shiny)
library(jsonlite)
library(httr)
library(plotly)
# Create a vector of municipalities in Latvia
municipalities <- c("Aglona", "Aizkraukle", "Aizpute", "Akniste", "Aloja",
"Alsunga", "Aluksne", "Amata", "Ape", "Auce", "Babite",
"Baldone", "Baltinava", "Balvi", "Bauska", "Broceni",
"Carnikava", "Cesvaine", "Ciblas", "Daugavpils", "Dobele",
"Dundaga", "Durbes", "Engure", "Ergli", "Garkalne",
"Grobinas", "Gulbene", "Iecava", "Ikskile", "Ilukste",
"Incukalns", "Jaunjelgava", "Jaunpiebalga", "Jaunpils",
"Jekabpils", "Jelgava", "Jurmala", "Kandava", "Karsava",
"Kegums", "Kekava", "Koceni", "Koknese", "Kraslava",
"Krimulda", "Krustpils", "Kuldiga", "Kumene", "Lielvarde",
"Ligatne", "Limbazi", "Livani", "Lizums", "Ludza", "Madona",
"Mazsalaca", "Naukseni", "Nereta", "Nica", "Ogre", "Olaine",
"Ozolnieki", "Pargauja", "Pasvalys", "Pelcku", "Plavinas",
"Preili", "Priekuli", " Priekule", "Rauna", "Rezekne",
"Riebi", "Riga", "Rujiena", "Sabile", "Salacgriva",
"Salaspils", "Saldus", "Saulkrasti", "Seda", "Sigulda",
"Skriveri", "Skrunda", "Smiltene", "Stopini", "Strenci",
"Talsi", "Tukums", "Valka", "Valmiera", "Vandzene",
"Vecpiebalga", "Vecumnieki", "Ventspils", "Viesite",
"Vilaka", "Vilani", "Zilupe")
# Create a Shiny UI
ui <- fluidPage(
titlePanel("Weather App"),
selectInput("municipality", "Select municipality", municipalities),
mainPanel(
tabsetPanel(
tabPanel("Current Weather", tableOutput("current_weather")),
tabPanel("Temperature Forecast", plotlyOutput("forecast_plot"))
)
)
)
# Define the function to get current weather data
get_weather_data <- function(municipality) {
api_key <- "c85d8912939742411f4756c70790238e" # your valid API key
url <- paste0("http://api.openweathermap.org/data/2.5/weather?q=",municipality,",lv&appid=",api_key,"&units=metric")
data <- httr::GET(url)
data <- httr::content(data)
return(data)
}
# Define the function to get forecast weather data
get_forecast_data <- function(municipality) {
api_key <- "44a8b47e94fd6388904950fd39d5b07d" # your valid API key
url <- paste0("http://api.openweathermap.org/data/2.5/forecast?q=",municipality,",lv&appid=",api_key,"&units=metric")
data <- httr::GET(url)
data <- httr::content(data)
return(data)
}
server <- function(input, output) {
weather_data <- reactive({
if (!is.null(input$municipality)) {
get_weather_data(input$municipality)
}
})
forecast_data <- reactive({
if (!is.null(input$municipality)) {
get_forecast_data(input$municipality)
}
})
#render current weather information
output$current_weather <- renderTable({
weather_data()
})
output$forecast_plot <- renderPlotly({
#Retrieve forecast data
forecast_data <- get_forecast_data(input$municipality)
# extract temperature and date-time data
forecast_temp <- forecast_data$list$main$temp
forecast_time <- as.POSIXct(forecast_data$list$dt, origin="1970-01-01", tz="UTC")
#create plot
plot_ly(x = forecast_time, y = forecast_temp, type = 'scatter', mode = 'lines+markers') %>%
layout(title = 'Temperature Forecast')
})
}
ui <- fluidPage(
titlePanel("Weather App"),
selectInput("municipality", "Select municipality", municipalities),
mainPanel(
tabsetPanel(
tabPanel("Current Weather", tableOutput("current_weather")),
tabPanel("Forecast Weather", plotlyOutput("forecast_plot"))
)
)
)
#server
server <- function(input, output) {
output$current_weather <- renderTable({
get_weather_data(input$municipality)
})
output$forecast_plot <- renderPlotly({
#Retrieve forecast data
forecast_data <- get_forecast_data(input$municipality)
# extract temperature and date-time data
forecast_temp <- forecast_data$list$main$temp
forecast_time <- as.POSIXct(forecast_data$list$dt, origin="1970-01-01", tz="UTC")
#create plot
plot_ly(x = forecast_time, y = forecast_temp, type = 'scatter', mode = 'lines+markers') %>%
layout(title = 'Temperature Forecast')
})
}
shinyApp(ui = ui, server = server)
The script starts by loading several libraries: shiny, jsonlite, httr, and plotly. These libraries are used to create the Shiny app, work with JSON data, make HTTP requests, and create interactive plots, respectively.
Next, the script defines a vector of municipalities in Latvia. This vector is used to populate the options in the dropdown menu in the app’s user interface.
The script then defines the user interface (UI) for the app using the Shiny function fluidPage()
. The UI includes a title panel, a dropdown menu for selecting a municipality, and a main panel with two tabs: one for displaying the current weather and another for displaying the temperature forecast.
The script then defines two functions to get the current weather and temperature forecast data for a given municipality. These functions use the httr
library to make HTTP requests to the OpenWeatherMap API and return the data in the form of a JSON object.
Finally, the script defines the server function that controls the behavior of the app. Inside the server function, the script uses the reactive()
function to create reactive objects for the current weather and temperature forecast data. These reactive objects are then used to update the output in the UI when the user selects a municipality from the dropdown menu. In particular, the tableOutput()
function is used to display the current weather data in a tabular format, and the plotlyOutput()
function is used to create an interactive plot of the temperature forecast data.